Robot acceleration
by Javantea
Sept 5, 2022
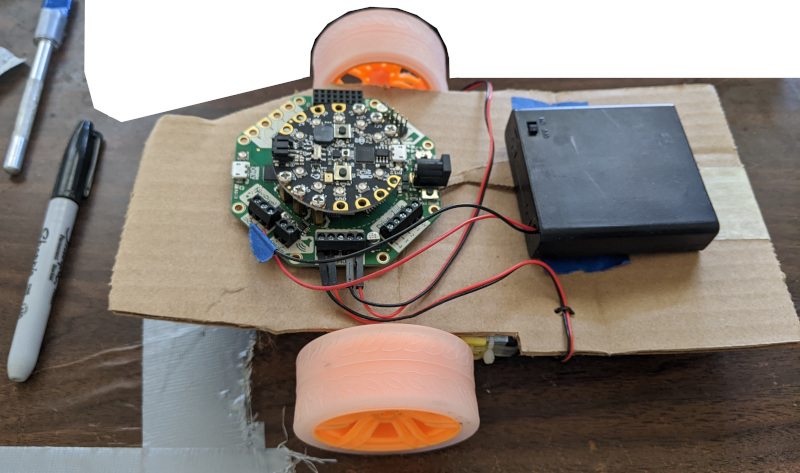
I have recently built a small robot as a test platform for an advanced robotics project. Huh? Let’s put the advanced robotics project aside and talk about what I actually have. I have a working robot that can detect hard collisions and the code is 120 lines of CircuitPython (not counting the libraries used to be clear). I can write a lot more code, but there’s no point to make it overly complicated until certain goals are met. Those goals: detect soft collisions, detect false-positive accelerations that are not collisions, and store information. While these may seem pretty easy to overcome, the thesis of this blog post is that they are not.
Skippable Introduction
Let’s start at the very start. When I was young I wanted a robot. I didn’t know why very well, but I knew that I did. I bought Handbook of Advanced Robotics from a used bookstore, a bunch of motors, balsa, wheels, and other RC stuff from my local hobby shop, and started building. After building quite a few uncontrolled cars, I realized that a lot of the robot lies in control. It did not occur to me until I reached university how much. I tried to build an ornithopter and after propulsion failed on that project, I went off to university and gave up on robots. As time went on I worked on electronics and realized that while I was better than average at soldering, I was particularly bad at finishing projects. Each project had an obstacle. Not only did I lack experience as a young person, I lacked the necessary support to complete projects of even modest difficulty.
Adabox
Enter Adabox 008. Adafruit is a very reputable electronics company which supplies kits and components at reasonable prices. I luckily started the Adabox subscription at 008 and got the robot parts (and a lot of other stuff) for $60 in 2017. I looked at the projects and found the rover project. I decided to do it and put it off. In 2018-2019 I tried building a robot with a Raspberry Pi, a motor hat, and two motors. It did not work. Why didn’t it work? Because in order to turn a wheel that is supporting the weight of a very, very light robot, a motor needs an incredible amount of torque. How much torque? More torque than the motors had. Where does one get torque? As a student of physics I’m well aware that torque is a trade off with speed. Using mechanical advantage one can convert speed (which the motors have a lot) to torque (which they have almost none). Mechanical advantage is the realm of machines like levers and a whole system of levers connecting a motor to a wheel is known as a pair of gears. You can use pulleys or other machines, but gears are definitely the right choice for most projects. But looking at the robot I had, I had nowhere to put a gear even if I had one. I needed custom hardware to mount such a system. Rods can be bought from the hardware store, but aluminum, plastic, or steel? Well, it turns out that you can buy those from the hardware store too, but I didn’t think it through. I now own a drill and so the custom robotics hardware is absolutely within my reach. I even took a class on welding in 2019, so I can technically build a robot in a welding class if I wanted to.
But who wants to build a robot out of metal? I went back to the Adabox and found the off-the-shelf motors with a gearbox suited for robots. The Adabox has the wheels. It was time to build a robot. I hot glued the motors onto a piece of cardboard, then hot glued the 3x AA battery case to the bottom of the robot, and then hooked it up. I programmed it in a few hours. It ran. There were a few issues. The 3x AA battery case is made for alkaline batteries which die when they run out of voltage. There’s no sense in making a robot and buying 20x alkaline AA batteries and go through them like I’m made of money. So I found my 4x AA battery case and put 4x eneloop batteries in it. It runs fine. If you’re curious why I couldn’t put 3x eneloop batteries in the 3x AA battery case, then you might not know that eneloop batteries (and most rechargable batteries in the AA form factor) charge to 1.2V while alkaline batteries supply 1.5V. If you try to supply a robot with 3.6V when it needs 4-5V, it will not work.
So the first few tests of the robot went quite well. Well enough that my posts to youtube were quite a hit.
I did a lot of work to try to figure out the correct value for collision detection, but essentially failed. Now we’re caught up. I put the robot on hold while other projects took my attention.
Last week I did a bunch of research and found that I’m pretty close to the part of the project where other people start getting creative.
Today I took some data on the test bench and wrote a bit of code to do the necessary analysis.
Code
import numpy as np
import matplotlib.pyplot as plt
= np.genfromtxt('accel5.txt', delimiter=' ')
a
0])
np.average(a[:,0.34817875
1])
np.average(a[:,0.27905393333333334
2])
np.average(a[:,10.122568999999997
0])
np.std(a[:,0.05937704541869834
1])
np.std(a[:,0.030664540153553403
2])
np.std(a[:,0.15099028149409702
0])
plt.plot(a[:,#[<matplotlib.lines.Line2D object at 0x7f248c5b56c0>]
plt.show()1])
plt.plot(a[:,#[<matplotlib.lines.Line2D object at 0x7f246461d330>]
plt.show()2])
plt.plot(a[:,#[<matplotlib.lines.Line2D object at 0x7f2464689e70>]
plt.show()
Data
When standing still and with no interference, we can expect a value of 0.34 m/s² ± 0.06 in the x direction, 0.28 m/s² ± 0.03 in the y direction, and 10. m/s² ± 0.15 in the z direction, depending on orientation. Why depending on orientation? Notice the 10. in the z direction. This is suspiciously close to 9.8 m/s², which is the acceleration of objects near the Earth. It’s not a coincidence. Unfortunately, the accelerometer is designed with a few applications in mind: detecting falling in gravity (at which point the acceleration goes to 0), and sensing orientation. Both of these goals are met by detecting gravity. However, that means that when standing still, it’s telling us that it’s accelerating, which is definitely not true. So instead of trusting the acceleration value, we instead depend on the change in acceleration. Change in acceleration (a₁ - a₀) tells us when the object actually accelerates. You can see from the graphs and the data that indeed when standing still, the values do not change drastically.
Issues
Running the robot we can clearly see that many collisions it will ignore when da² ≥ 144. That coincides with a change in acceleration of 12 m/s². (Note: It doesn’t make sense to call it m/s³ because we are just subtracting two accelerations: a₁ - a₀, not dividing by time.) In the opposite direction, when reversing over very flat terrain, it will cause itself to vibrate due to the elastic nature of the cardboard and detect a collision. By reinforcing the cardboard structure of the robot this effect can be reduced, but any collision can weaken the structure.
Clearly the solution to the weak structure is to buy bronze, brass, steel, aluminum, wood, or plastic from a hardware store. Of these options, plastic is my favorite but is also fairly difficult to find. Information on my eventual success in this direction will be posted in updates.
Logging to flash is extremely slow, which changes the result of the many experiments. That means that any logging has to be done while the roboticist follows the robot with a laptop. This should not be the case, so it’s possible that this can be fixed.
Writing software for the robot is pretty easy and fun. Lack of memory and multitasking is a major concern for writing more complex software. Unfortunately switching to a raspberry pi and pi motor hat will involve rewriting some or all of the software. Luckily, the software is small and simple to rewrite. It would be possible to connect the Crickit to the raspberry pi by USB cable, but that would waste electricity which is in even higher demand when using a raspberry pi.
Conclusion
In my endeavor to build a advanced robotics project, I find myself with a small and simple robotics project. I have overcome simple obstacles and created something. Along the way we’ve learned about physics, engineering, software, and human life. I hope that the journey of this robot has inspired you to learn the easy projects.
Permalink-
Leave a Reply
Comments: 0
Leave a reply »